虽然不是美剧的忠实爱好者,但是还是关注了一些比较有意思的美剧,很多时候由于比较忙或者忘记了去查看美剧更新,错过了这些精彩剧集。
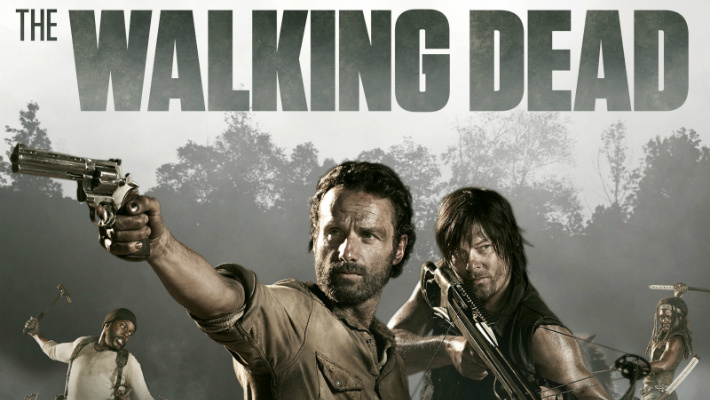
这里用python+VPS实现了在线检查美剧页面是否有剧集更新,有的话就发邮件提醒。这里以《行尸走肉》和《生活大爆炸》为例。
依赖
检查更新
这部分主要就是简单的html页面解析,将相对应的剧集名称存在txt中,每次解析一次网页,并将得到的结果与txt中的结果进行比较,如果不同,则有更新,返回最新的剧集和百度网盘地址
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75
|
import requests from lxml import etree import os import smtplib from email.mime.text import MIMEText import time
def send_email(series, download_url): _user = "xxx@163.com" _pwd = "xxxx" _to = "xxxxxxxxxxxxx"
content = series + ' 已更新' + '\n\n' + '百度网盘地址: ' + download_url msg = MIMEText(content) msg["Subject"] = '剧集更新提醒' msg["From"] = _user msg["To"] = _to
s = smtplib.SMTP("smtp.163.com", timeout=30) s.login(_user, _pwd) s.sendmail(_user, _to, msg.as_string()) s.close()
def check_series_update(url): r = requests.get(url) page = etree.HTML(r.text) hrefs = page.xpath(u"//a") current = [] for index, href in enumerate(hrefs): txt = href.text if txt and 'SCG' in txt: current.append(txt.encode('utf-8')) current.append(hrefs[index+1].attrib['href'])
curText = ''.join(current)
series = url.split('/')[-1] series_file = series[0:-5] + '.txt'
if os.path.isfile(series_file): f = open(series_file) lastText = f.read() f.close()
if curText == lastText: print 'No update' else: f = open(series_file, 'w') f.write(curText) f.close() send_email(current[0], current[1]) else: f = open(series_file, 'w') f.write(curText) f.close()
def check_update(urls): while True: for url in urls: check_series_update(url) time.sleep(5)
if __name__ == "__main__": urls = ["http://www.ttmeiju.com/meiju/The.Big.Bang.Theory.html", "http://www.ttmeiju.com/meiju/THE.Walking.Dead.html"] check_update(urls)
|
vps运行
将脚本放在服务器上,利用screen
指令创建新窗口,在该窗口中运行脚本,即可实现实时监控
相关参考内容
由于可以直接获取到百度网盘的链接,后期考虑看可否直接将该链接中的内容保存到自己的百度网盘指定目录,那样就更加方便了